Situs Toto : Trend Yang Tidak Bisa Di Stop Situs toto online telah mengalami peningkatan popularitas yang tajam dalam beberapa tahun terakhir terutama selama masa pandemi. Semakin banyak orang terutama generasi muda memilih untuk memasang toto mereka secara online. Omset judi online mencapai 20 miliar USD di Indonesia selama tahun 2023. Ini angka yang sangat …
Menang Dan Profit Di Toto Online Dengan Metode Ini
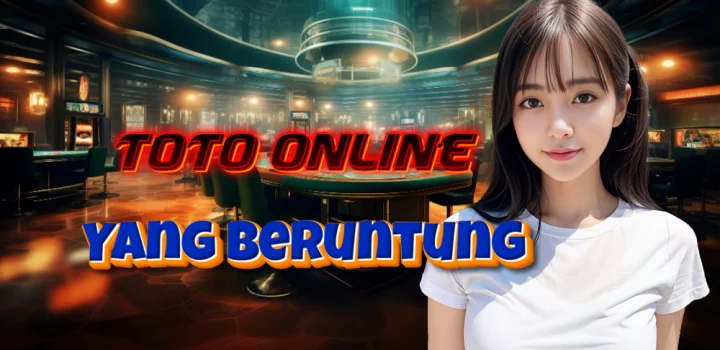